How to Allow All Domains for Image Configuration in Next.js?
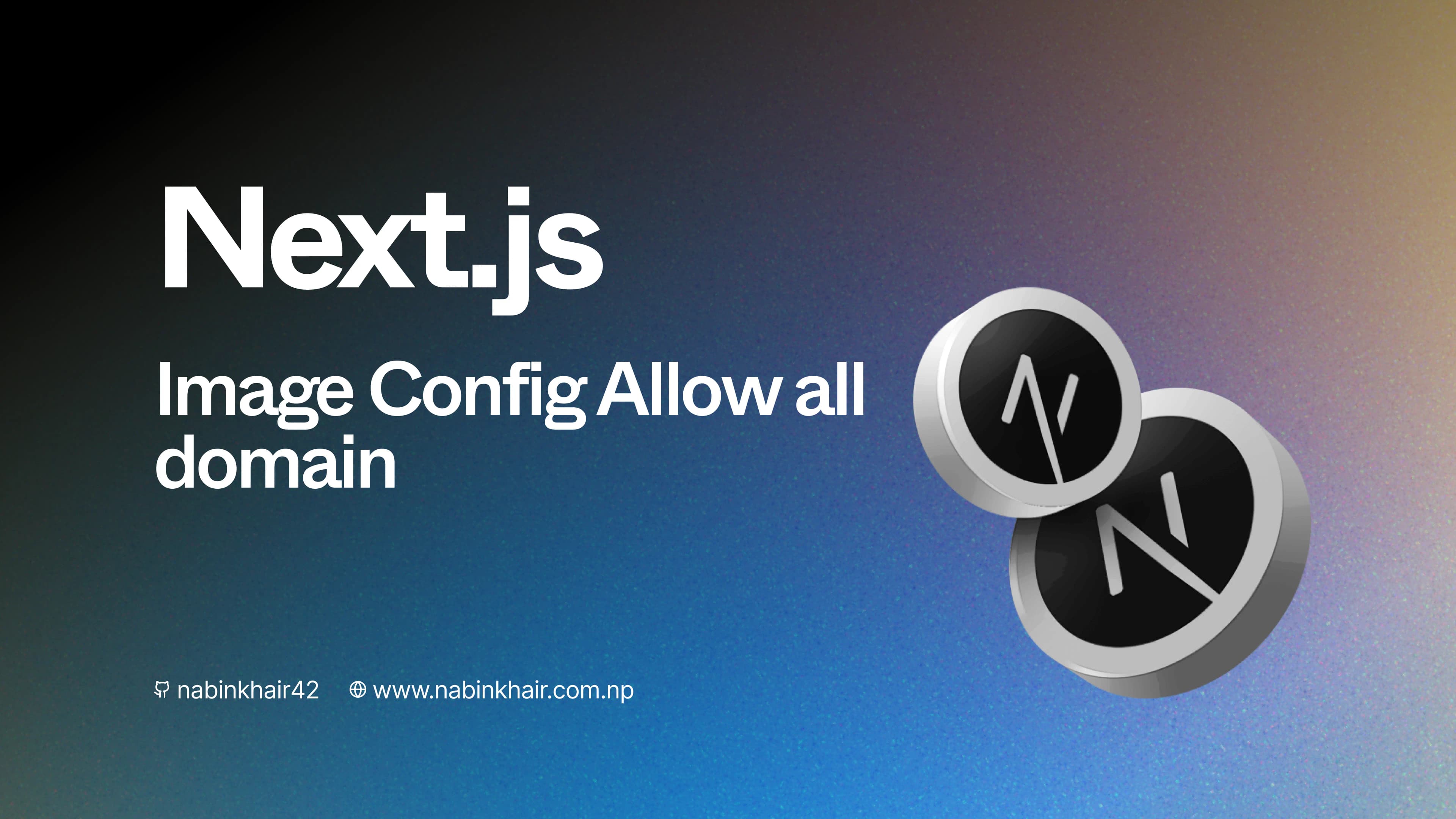
Introduction
When using the Image
component in Next.js to load images from external URLs, you must configure your project to allow these domains. This step ensures secure handling of remote images while optimizing performance. In this guide, we’ll explore how to allow custom domains and all domains for external images in Next.js.
Allowing a Custom Domain
To allow images from a specific domain (e.g., images.unsplash.com
), follow these steps:
Step 1: Update next.config.js
Open your next.config.js
file and add the following configuration:
module.exports = {
images: {
remotePatterns: [
{
protocol: 'https',
hostname: 'images.unsplash.com',
},
],
},
};
Step 2: Restart the Development Server
After saving your changes, restart the server:
npm run dev
Step 3: Verify the Configuration
Load your application and check that external images from the configured domain are displaying correctly.
Common Error:
If you encounter an error, ensure that you’ve used hostname
instead of host
. This is a common mistake that can prevent the configuration from working.
Allowing All Domains
If your application needs to support images from any domain, you can use a wildcard configuration. This approach is less secure but useful for testing or handling dynamic domains.
Step 1: Modify next.config.js
Update your next.config.js
file to include the following wildcard configuration:
module.exports = {
images: {
remotePatterns: [
{
protocol: 'https',
hostname: '**',
},
],
},
};
Step 2: Restart the Development Server
Save the file and restart the server to apply the changes:
npm run dev
Step 3: Test the Setup
Load your application and verify that images from any secure domain are displaying correctly.
Pro Tip:
Use this configuration only when absolutely necessary, as it is less secure than specifying individual domains. Always restart the development server after changing next.config.js
to ensure the updates take effect.
Conclusion
Configuring image domains in Next.js is essential for secure and efficient handling of external images. By specifying trusted domains or using a wildcard for all domains, you can customize your application’s image-handling capabilities.
Key Takeaway:
While allowing specific domains is recommended for most scenarios, enabling all domains can be useful during development or for specific use cases. Tailor your configuration to your project’s needs for optimal results.
By following this guide, you can ensure seamless integration of external images in your Next.js applications.